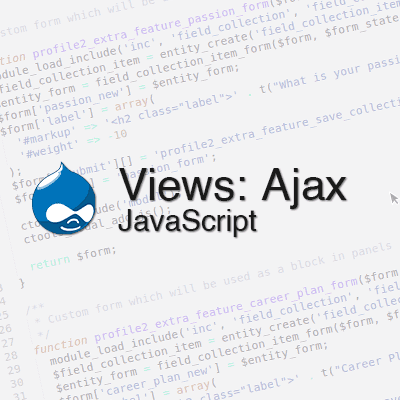
Drupal: Views Ajax
Most of us know that Views module has its own Ajax functionality. If we enable the ajax checkbox in the view settings, a bunch of Ajax-related JavaScript files will be loaded for us on our views' page.
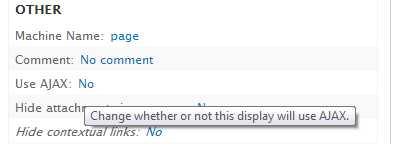
One of the files which will be loaded is:
/views/js/ajax_view.js
If we drill down this file and analyze it, we will understand some of the Views' ajax functionality. Lets take a case and see how we can reuse Views' Ajax.
Reloading a View with specific arguments via Ajax:
Lets say we have 2 views on the same page, for instance we use Panels and we have a left-side view and a content view. The content view is empty on the first page load, but when user selects something in the left-side view we want to reload dynamically the content view with specific arguments passed to it.
First idea that came to my head is to use my custom JavaScript script that will take some arguments onclick from the left-side view row, will make a custom Ajax call to one of my modules' callback, that will use views_embed_view to render the new output and use drupal json to output it back to the page. Then I'd simply replace the contents of the view and we're done.
But I kept thinking that declaring a custom callback, processing functions (and where are callback functions there are access functions) and custom ajax calls is a wrong way, because Views module handles all of those by default.
All of the Ajax-enabled views instances are stored in Drupal.views.instances and all of them are of Drupal.views.ajaxView type.We can use these instances to make the drupalish ajax calls which will update the views in the right way.
But first we need to inject our JavaScript into a specific view. We can use preprocessing functions but I'd like to use my .module file and hook_views_pre_render(). One interesting thing to notice here, make sure your JavaScript file is loaded after ajax_views.js, this is why we used JS_THEME group.
/** Â * Implements hook_views_pre_render(). Â */ function campaigns_feature_views_pre_render(&$view) { if ($view->name == 'active_campaigns' && $view->current_display == 'panel_pane_sidebar') { drupal_add_js(drupal_get_path('module', 'campaigns_feature') . '/js/active_campaigns.ui.js', array('group' => JS_THEME)); } }
Once the JavaScript is loaded, we can proceed to implementation.Â
(function($) { Drupal.behaviors.activeCampaigns = { // First we use the settings object to get the views' details var views = settings.views.ajaxViews; Â // view_dom_id is an unique value, it's unique per view and per pageload var view = view_dom_id = view_name = undefined; for (var viewsName in views) { if (views.hasOwnProperty(viewsName)) { view = views[viewsName]; // I used view_display_id to actually identify my view // and store it in the view variable if (view['view_display_id'] == "pane_active_camp_users") { view_name = viewsName; view_dom_id = view["view_dom_id"]; } } } Â // Then we use view_name (which is views' key that contains unique view_dom_id) // to do our manipulations var instance = Drupal.views.instances[view_name]; // Then we pass through all of the left-side views items var $this = $(element), viewData = {}, // Load the argument (it's actually hidden in the view, not excluded) instance.settings['view_args'] = nid; // This is the way we update the viewData object with defaults and // later with overridden values // Create the Drupalish ajax call }); Â } }
You can tweak viewData and/or instance.element_settings how you want (for instance to load the view onmouseover event) to achieve the right functionality.
And we all can forget about writing custom callbacks and just re-using Views' Ajax functionality.
💡 Inspired by this article?
If you found this article helpful and want to discuss your specific needs, I'd love to help! Whether you need personal guidance or are looking for professional services for your business, I'm here to assist.
Comments:
Feel free to ask any question / or share any suggestion!